Although C is 5 decades old, it is still among the most in-demand skills. Even though modern languages like Python, Golang, Go and Rust are popular, they are still way behind in building a legacy like that of the C programming language.
Learning C is, although, not necessary to build a career in software development these days, it is an important skill to have to build a solid foundation in programming. So, here, we will be discussing some of the most-asked C interview questions.
Most Frequently Asked C Interview Questions
Easy C Interview Questions
1. List down the features of the C programming language.
The following are the important features of the C language:
- Easy to understand.
- Structured language.
- It has a rich function library.
- It offers dynamic memory management.
- You can free the allocated memory as per the requirement.
- It is used for developing system applications like drivers and kernels.
- It supports high-level language features.
- It can easily and efficiently adopt new features.
2. What is the difference between calloc() and malloc()?
These two functions facilitate dynamic memory allocation in C. Following are the points that differentiate between these two functions:
calloc | malloc |
It will load all the memory locations assigned with 0 value. | It will not load all the memory locations assigned with a 0 value. It contains garbage value. |
You can assign multiple blocks of memory using calloc. | You can only create a single block of memory of the desired size. |
It is more secure. | It is less secure. |
The calloc() function returns the beginning address and sets it to zero before allocating the memory. | The malloc() function does not set the address to 0 but just returns the starting address. |
It can do memory initialization. | It cannot do memory initialization. |
3. Is it possible to generate a customized header file in C?
Making a new header file is both possible and simple in C. You can make a file containing function prototypes a header file and use it throughout C code. Simply, put the file’s name in the “#include” section.
4. Differentiate between actual parameters and formal parameters.
Actual Parameters: These parameters are the arguments passed to a function call. The calling function determines these parameters. They are listed in the parameter list of a subprogram call. It is not required to specify the data type in the argument itself.
Example: add(x,y);
x and y are actual parameters here.
Formal Parameters: These are the variables or expressions that are listed in the parameter list of a subprogram definition. It is compulsory to mention the data type of the received value. The scope of formal arguments is constrained to the function declaration in which they are used.
Example: int diff(int x, int y)
{
//Code inside function
}
x and y are Formal Parameters here
5. Define the scope of the variable.
A scope is a region within which a variable can have its usage and existence. A variable is not accessible if it’s accessed beyond its scope. The scope of a variable can be of two types:
1. Local Variables: These variables are declared within a function or block. Only statements included within that function or code block are permitted to use them.
Example:
#include <stdio.h>
int main () {
// local variable declaration
int x, y;
int sum = 0;
// actual initialization
x = 45;
y = 30;
sum = x + y;
printf (“x = %d, y = %d and sum is = %d\n”, x, y, sum);
return 0;
}
2. Global Variables: These variables are declared outside of the function or block. The global variable can have its value altered by any function. It can be used for all functions but it has to be declared at the beginning of the block.
Example:
#include <stdio.h>
// global variable declaration
int globSum;
int main () {
// local variable declaration
int x, y;
// actual initialization
x = 18;
b = 67;
globSum = x + y;
printf (“x= %d, y = %d and Sum = %d\n”, x, y, globSum);
return 0;
}
6. What type of language is the C programming language?
In the C programming language, everything is in the form of a function. Hence, C is a procedural programming language.
Intermediate C Interview Questions
7. Does C language support function overloading?
Most object-oriented languages, including Java, Python, and C++ support function overloading, however, C does not. Nonetheless, one may indirectly achieve function overloading in the C programming language. The first strategy goes like this:
You can pass a void * pointer as an argument to a function. Pass another argument in the same function that would tell the actual data type of args1 passed.
int foo(void * arg1, int arg2);
8. How to do dynamic memory allocation in C?
The process of allocating memory to a program and its variables during execution is known as dynamic memory allocation. There are three functions in the dynamic memory allocation process to allocate memory, and one function to release memory that has been used. These functions are present under the <stdlib.h> header.
You can think of dynamic allocation as a method of utilizing heap memory where we can change the size of a variable at any time while a program is running using library functions.
1. malloc() – Used to allocate memory
Syntax:
ptr = (cast-type*) malloc(byte-size);
2. calloc() – Used to allocate memory
Syntax:
ptr = (cast-type*)calloc(n, sizeOfElement);
3. realloc() – Used to allocate memory
Syntax:
ptr = realloc(ptr, eleNewSize);
4. free() – Used to deallocate the used memory
Syntax:
free(ptr);
9. What are pointers in C?
Pointers are used to keep the address of a variable or memory location. The major goals of the pointer are to decrease execution time and boost memory efficiency. Following are some of the uses of pointers:
- You can return multiple values from a function.
- You can pass arguments by reference.
- To perform dynamic memory allocation.
- You can easily implement data structures using pointers.
- You can use it for accessing array elements.
10. Explain three different pools of memory in C language.
There are three separate memory pools in C:
- static: permanent global variable storage for the duration of the program.
- stack: regional temporary storage
- heap: a dynamic storage system
- Static: The reverse of the stack is called a heap. The heap, also known as the “free store,” is a sizable pool of dynamically usable memory. You should directly allocate (using functions like malloc) and deallocate (e.g., free) this memory because it is not automatically managed. There are typically no limitations on the size of the heap (or the variables it creates), unlike the stack. You can access the variables created on the heap anywhere within the program.
- Stack: The variables that you use inside a function, including the main() function, are part of the stack memory. It is a “Last-In, First-Out,” or LIFO, structure. The stack is “pushed” every time a function declares a new variable. Then, after a function has completed its execution, all of its stack-based variables are erased, freeing up the memory they were using. The “local” scope of function variables results from this.
- Heap: Global variables and variables created with the static clause are often stored in static memory, which lasts the whole duration of the program. This variable requires 4 bytes of memory on various systems. There are two possible sources for this memory. A variable is regarded as global if it is defined outside of a function, which means it can be accessed from anywhere in the program. Global variables are static, and the entire program only has one copy of each.
11. Briefly explain the concept of call by value and call by reference in C.
Call by value:
- The formal parameters receive a copy of the value of the actual parameters. In other words, the call by value technique uses the variable’s value when calling the function.
- The formal parameter cannot change the value of the actual parameter.
- Since the value of the actual parameter is duplicated into the formal parameter, separate memory is allotted for each.
Example:
#include<stdio.h>
void main() {
#include<stdio.h>
void swap(int a, int b)
{
int temp = a;
a = b;
b = temp;
}
int main() {
int a = 70;
int b = 30;
printf(“The numbers before swapping a and b %d %d \n”,a, b);
swap(a, b);
printf(“The numbers after swapping a and b %d %d \n”,a, b);
return 0;
}
Call by reference:
- The actual parameter for the function call is the address of the variable.
- Since the address of the actual parameters is passed, the value of the actual parameters can be changed by altering the formal parameters.
- Both formal parameters and actual parameters have a comparable memory allocation. The value stored at the address of the actual arguments is the starting point for all actions within the function, and the updated value is also saved there.
Example:
#include<stdio.h>
void swap(int *a ,int *b)
{
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int a = 80;
int b = 50;
printf(“The numbers before swapping a and b %d %d \n”,a, b);
swap(&a, &b);
printf(“The numbers after swapping a and b %d %d”,a, b);
return 0;
}
Advanced C Interview Questions
12. Tell the difference between const char* p and char const* p?
The pointer const char* p points to a const char.
The pointer char const* p points to a char const.
Const char and char const are the same in C language.
13. What is the difference between double quotes (“”) and angular braces (>) in the header of the C language?
When a header file is enclosed in double quotations (“), the compiler first looks for that specific header file in the working directory. If not, it looks like the inclusion path for the file. However, the compiler only looks in the working directory for the specific header file when the header file is enclosed in angular brackets (>).
C Programming Interview Questions
14. How can you generate random numbers in the C programming language?
You can easily generate random numbers using the rand function. Following is the code to generate a random number:
#include<stdio.h>
#include<stdlib.h>
int main()
{
int x,y;
for(x=1;x<=10;x++)
{
y = rand();
printf(“%dn”,y);
}
return 0;
}
15. Print “Hello World” without a semicolon in C language.
#include<stdio.h>
void main()
{
if(printf(“hello world”)){}
}
16. Find the output of the code snippet below.
#include<stdio.h>
int main()
{
int n;
for(i = 5; i!=0; i–)
printf(“i = %d”, i–);
getchar();
return 0;
}
Output: Infinite loop as the value of ‘i’ is never 0 when the i!=0 condition is checked.
17. Write a C program to check if a number is a palindrome or not.
#include <stdio.h>
int main()
{
int num, rev = 0, remainder, number;
printf(“Enter an integer: “);
scanf(“%d”, &num);
number = num;
while( num!=0 )
{
remainder = num%10;
rev = rev*10 + remainder;
num /= 10;
}
if (number == rev)
printf(“\n%d is a palindrome\n”, number);
else
printf(“\n%d is not a palindrome\n”, number);
return 0;
}
18. Write a C program to check if the number is prime or not.
#include <stdio.h>
main() {
int num, i, count = 0;
printf(“Enter any number:”);
scanf(“%d”, &num);
//prime numbers have only factors (1 and itself).
//count the factors of number from 1 to num.
//iterate from 1 to num
for (i = 1; i <= num; i++) {
if (num % i == 0) {
count++;
}
}
//if count is 2 then its prime number
if (count == 2) {
printf(“It is a Prime number”);
}
else {
printf(“It is not a Prime number”);
}
return 0;
}
19. Describe “typedef” in C language.
The keyword typedef defines an alias for an existing type in C programming. No matter if the name is for a structure declaration, function parameter, or integer variable, typedef will abbreviate it.
Syntax:
typedef <existing-type> <alias-name>
Example:
typedef long long ll
20. Describe the l-value and r-value in C.
A data value that is kept in memory at a specific location is referred to as an “r-value.” A value cannot be assigned to an expression that has an r-value, hence this expression can only occur on the right side of the assignment operator (=).
A memory location that is used to identify an object is referred to as an “l-value.” Either the left or right side of the assignment operator (=) contains the l-value. In many cases, the l-value is utilized as an identification.
21. Differentiate between struts and union keywords in C language.
Structure: A structure is a special form of data. Multiple members of various data kinds can be contained within a single structure. The components of a structure are kept in consecutive memory regions and are always accessible. In a structure, each data object is a member or field.
Syntax:
struct [name_of_structure]
{
type member_1;
type member_2;
. . .
type member_n;
};
Example:
struct employee
{
int empno;
char empname[50];
string empphone;
};
Union: It is a user-defined data type. It is similar to the structure but each component starts at the exact same place in memory. In the precise region of memory, the union brings together items of various data types. Although a union can have numerous members, only one of them can contain a value at once. The amount of storage space allotted for the union variable is equal to the total amount of space needed for the union’s most salient data member.
Syntax:
union [name_of_union]
{
type member_1;
type member_2;
. . .
type member_n;
};
Example:
union Employee {
char empname[32];
int empage;
string empdept;
};
Conclusion
Practically all other languages are based on the fundamental language C. All other programming languages are built upon it. It is a widely used and well-liked language for creating system applications. Despite the fact that other languages now outnumber it in popularity, it is still one of the most widely used programming languages.
Because C is a fundamental language, it is crucial to have at least a basic understanding of it when applying for a job. With this blog, we aimed to provide a series of frequently asked C questions that would give you confidence in the language’s fundamentals.
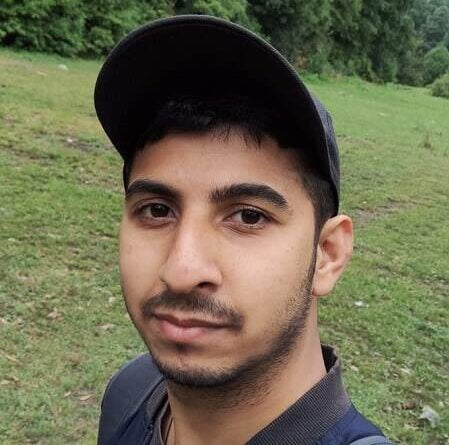
Aditya is a seasoned JavaScript developer with extensive experience in MEAN stack development. He also has solid knowledge of various programming tools and technologies, including .NET and Java. His hobbies include reading comics, playing games, and camping.